Raspberry Pi Microproject
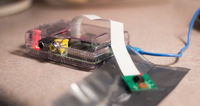
I've had a Raspberry Pi for some time, and I'm still wondering what I should do with it. Occasionally I have an idea and I go to start it up and ... realize I've forgotten the password.
Oops.
Well, this blog post isn't about that, but I'll mention a fix at the end just for my own reference, along with some links to a Raspberry Pi starter kit & camera modules.
What I need is for a freshly-booted, headless, keyboardless Raspberry Pi to somehow tell me its IP address.
This microproject took me about an hour to complete, including recovering the password. The idea stems from the fact that when I connect the Pi to a network that dispenses IP addresses using DHCP, I can't guess what address it'll get. Sure, on networks I control, I can pin the MAC Address of the Pi to a specific IP - but I don't always control the network.
Enter: Twilio.
Before deciding to try Twilio, my first thought was "I know! I can just use email." Unfortunately - or, from a network administration standpoint, extremely fortunately - some firewalls block SMTP Port 25, so the Pi sometimes can't send email.
Twilio provides an API to send text messages from your own scripts. Being a web-based API, any standard network should grant you permission to communicate with the Twilio servers.
Of course, being a company, Twilio is not free to use. I was able to use a trial account to test this out, but normally there are small fees associated with obtaining an SMS number & sending each SMS message.
My Pi is running Raspbian Linux, which is a variant of the Debian operating system. This means I can run "sudo apt-get install curl php5 php5-cli php5-curl" to get PHP going, then install PHP's Composer package manager to start building a command line PHP script. If you're more comfortable using a different language, it's just a matter of sending a properly-crafted XML request to the Twilio server. Many languages even have a Twilio SDK that makes it much easier to get going. I just happen to like PHP, and I like using Composer because it lets me quickly find and integrate libraries like this SDK.
I ran "composer init"
in the folder /home/pi/SmsProject/
, accepted most of the defaults, said "no" to the dependency searches, and gave it the ever-so-plain name of "beryllium/sms".
Then I ran "composer require twilio/sdk"
to pull in the latest version of the Twilio PHP SDK.
After that, I needed to write a short PHP script (sms.php
) to pull it together. In fact, most of it was copied directly from the Twilio documentation.
#!/usr/bin/env php
<?php
require __DIR__ . '/vendor/autoload.php';
// retrieve the desired SMS payload from Standard Input
// (e.g., a unix pipe)
$smsBody = file_get_contents('php://input');
// set your AccountSid and AuthToken from www.twilio.com/user/account
$AccountSid = "ACXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX";
$AuthToken = "YYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYY";
$client = new Services_Twilio($AccountSid, $AuthToken);
$message = $client->account->messages->create(
array(
"From" => "YYY-YYY-YYYY", // Your Twilio number
"To" => "XXX-XXX-XXXX", // Your cell number
"Body" => $smsBody,
)
);
// Display a confirmation message on the screen
echo "Sent message {$message->sid}";
Then I did something strange. I switched languages. It wasn't strictly necessary, but based on past experience it did seem to be the smarter choice for the tasks ahead.
I wrote a Bash shell script to determine the current IP address of the Pi (if any) and send this information to the sms.php
script using Unix Pipelines. If you've never worked with Pipes before, you should look into them - they can be a great way to string small commands together to build useful and creative combinations of tools.
Here's what the shell script (/home/pi/bin/online_alert
) looked like:
#!/bin/bash
# give the system enough time to connect to WiFi
sleep 60
# try to grab the IP
CURRENT_IP=`ip route get 8.8.8.8 | awk 'NR==1 {print $NF}'`
# exit if we don't have an IP
if [ -z "$CURRENT_IP" ]
then
exit
fi
echo "Bubastis is ONLINE: $CURRENT_IP" | /home/pi/SmsProject/sms.php
In order to get it to execute on system boot, I had to mark both scripts as executable and add a line to the bottom of /etc/rc.local
:
# marking the scripts as executable:
$ chmod a+x /home/pi/bin/online_alert
$ chmod a+x /home/pi/SmsProject/sms.php
# adding to the bottom of /etc/rc.local:
/home/pi/bin/online_alert &
The ampersand at the end tells it to launch in a subprocess, which should prevent the script from interfering with the boot sequence in the event of a failure.
Whenever I plug in my Raspberry Pi, I get this text a short while later:
Now I just need to pay for my account.
Useful Links:
Raspberry Pi Starter Kit and Cameras:
One of my other Pi/Twilio ideas involves sending MMS texts containing images from the Pi camera module, either as a security system or a nature cam. I've picked up a plain Pi camera module from a retailer called SainSmart (found on Amazon), but eventually I might get the "NoIR" camera that can take photos and video in the infrared spectrum. Here are some Amazon links for the starter kit and different camera options:
Recovering a Forgotten Raspberry Pi Password:
- Remove the SD card and pop it into another machine
- Make a backup of the
cmdline.txt
file. - Now, edit
cmdline.txt
. The file only contains one line. Addinit=/bin/sh
to the end. - Save the file and return the SD card to the Raspberry Pi
- Boot the Pi. The sequence should stop before it asks for a login. If it looks like it's
hung or otherwise not responding, hit the Enter key - when you end up on a line with a
plain
#
prompt, you're ready to start. - Run the
mount
command to try and find the/
device - If the
/
device is read-only, usemount -o remount,rw {the / device} /
to flip it to Read-Write mode. - Run
passwd pi
to change your password. - Shut down the pi (
shutdown -h now
) - Remove the
init=/bin/sh
change fromcmdline.txt
using another machine (overwriting it with the backed-upcmdline.txt
is another way to undo our change)
If any of the commands fail, you may have to put sudo
before them.
Published: January 29, 2016
Tags: coding, dev, development, command-line, howto, administration, composer, starter-kit, password-recovery